Today I thought to start documenting my experiments with Arduino and Unity.
In this blog I will explain how to use Arduino to send input to your Unity game. It’s going to be a very simple example where two buttons will be used to move an object in Unity.
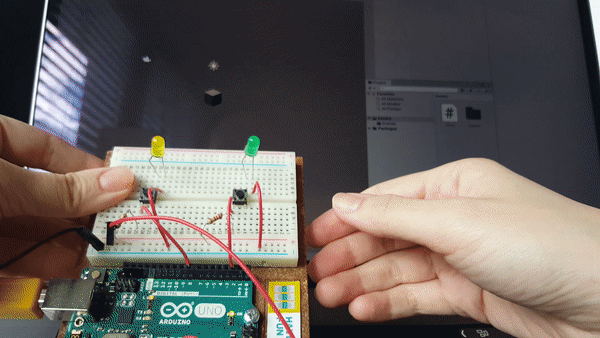
Code is available on GitHub.
Requirements:
- Unity
- Arduino Uno, 2 LEDs, 2 Push Buttons, wires, breadboard
What you will learn:
- How to send input from Arduino to Unity
Language:
- C# in Unity
- C/C++ functions for Arduino IDE
1. Play with Arduino
This is the schema that I have created for my experiment. For the creation of the schema Fritzing is a very easy online tool that allows you to create simple schema like this one by using drag and drop functionality.
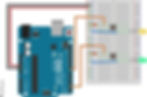
Use the Arduino IDE and plug-in your breadboard and use the following script.
const int pin_button1 = 8; // yellow-left
const int pin_button2 = 2; // green-right
void setup() {
Serial.begin(9600);
pinMode(pin_button1, INPUT);
pinMode(pin_button2, INPUT);
}
void loop() {
// yellow-left button is pushed
if(digitalRead(pin_button1)==LOW){
//Serial.println("yellow-left");
Serial.write(1); // Unity will read this value
Serial.flush();
delay(20); // This delay is important - 20 should be enough for a smooth transition.
}
// green-right button is pushed
if(digitalRead(pin_button2)==LOW){
//Serial.println("green-right");
Serial.write(2); // Unity will read this value
Serial.flush();
delay(20);
}
}
Important: When you plug-in your Arduino, make sure to choose the correct Port. Tool --> Port --> Select your port.
If everything goes well you should be able to see the yellow LED lighting when you press the left button and vice versa, if you click on the right button you should see the green led to light up.
Once everything is working let’s open Unity.
2. Create a Unity project
You should be able to create a new project. (I am currently using: 2020.1.1f1 Version)
Important: Change the setting of the player by going into Edit --> Project settings --> Player --> API Compatibility Level --> .NET 4.x

First, create an object cube by going to GameObject -> 3DObject -> Cube.
Secondly,you can import or create a new C# script by following this code. When you create a new serial port you should include the port name (COM port in Windows). On a Mac an easy way to do this is to go on the command line and use
ls /dev/tty.*
to list all the possible port available.
Make sure to name your C# file the same as your class name. In this case "Move".
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO.Ports;
// make sure to name your C# file the same as your class name. In this case Move.cs
public class Move : MonoBehaviour
{
// change your serial port
SerialPort sp = new SerialPort("/dev/tty.usbmodemxxxxxx", 9600);
// Start is called before the first frame update
void Start()
{
sp.Open();
sp.ReadTimeout = 100; // In my case, 100 was a good amount to allow quite smooth transition.
}
// Update is called once per frame
void Update()
{
if (sp.IsOpen){
try{
// When left button is pushed
if(sp.ReadByte()==1){
print(sp.ReadByte());
transform.Translate(Vector3.left * Time.deltaTime * 5);
}
// When right button is pushed
if(sp.ReadByte()==2){
print(sp.ReadByte());
transform.Translate(Vector3.right * Time.deltaTime * 5);
}
}
catch (System.Exception){
}
}
}
}
Once you have created the script, now you can add it to the object (cube) by drag-and-drop it on the object properties.
3. Play with both
Now, everything should be working. If you click on PLAY in Unity you are able to see the preview of the game and by clicking on the buttons you can see the cube moving from left to right.
Code is available on GitHub.
Conclusion
This can be applied to many transformation. In this simple case we change the position of the object but it can be applied to many different properties.
Useful Tutorials: